Add custom 3D objects
Sometimes it's useful to add custom content to the 3D scene to model data Reveal doesn't
support or to customize the 3D environment. Reveal supports this by using the
Cognite3DViewer.addObject3D
-function which accepts ThreeJS objects.
Reveal uses an "implicit lighting model", i.e. no lights are added to the scene. If you need lights this needs to be added to the viewer scene if the added objects isn't "unlit".
Adding markers to the scene on clicks
The following example reacts to clicks in the scene and adds markers to the positions clicked.
Load external GLTF content
GLTF is a commonly used 3D format for content delivery - think of it as the JPG of 3D.
GLTF is supported by ThreeJS and can easily be added to Reveal using GLTFLoader
.
GLTF is great for creating custom 3D content with high fidelity, and is supported by most 3D design software,
e.g. Blender. There's also several services for generating GLTF content (e.g. ElevationAPI)
and market places for 3D models for download (e.g. Sketchfab and Turbosquid) - allowing you
to quickly build "digital worlds" with Reveal.
In the following example, a GLTF model is loaded and placed in the clicked positions in the CAD model. The 3D model is from Grand Dog Studio on Sketchfab under the CC 4.0 license.
Add environment
To make a model feel more natural it might be useful to add an environment. The following example adds sky and a sea effect for the example oil rig.
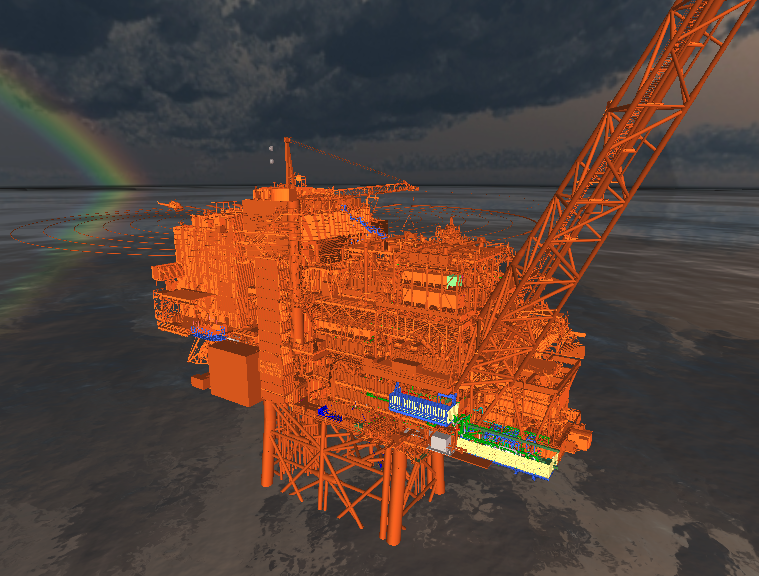
Below you'll find an interactive example, but first lets walk through the different parts. See the bottom of this page for an interactive example.
First, let's encapsulate the model in a large sky-textured sphere to create an illusion of skies. The sphere map is available from Free HDR - EXR SKIES-blog.
const modelCenterAtSeaLevel = model
.getModelBoundingBox()
.getCenter(new THREE.Vector3());
modelCenterAtSeaLevel.y = 0.0;
// Skybox
const skyBox = new THREE.Mesh(
new THREE.SphereGeometry(3000, 15, 15),
new THREE.MeshBasicMaterial({
side: THREE.BackSide,
// Skybox texture from http://freepanorama.blogspot.com/2010/01/sky7-spherical.html
map: new THREE.TextureLoader().load(urls.skyUrl),
})
);
skyBox.position.copy(modelCenterAtSeaLevel);
viewer.addObject3D(skyBox);
This looks a bit better, but the oil rig appears to be floating mid-air at this point. Lets add an ocean. A plane is rendered at the sea level.
// Water
const waterGeometry = new THREE.PlaneGeometry(6000, 6000, 63, 63);
const material = new THREE.MeshBasicMaterial({
map: new THREE.TextureLoader().load(
'https://threejs.org/examples/textures/water.jpg',
(texture) => {
texture.wrapS = texture.wrapT = THREE.RepeatWrapping;
}),
color: { value: new THREE.Color(0x0099ff)},
side: THREE.DoubleSide
});
const water = new THREE.Mesh(waterGeometry, material);
// Make the ocean horizontal
water.rotation.x = -Math.PI / 2;
water.position.copy(modelCenterAtSeaLevel);
viewer.addObject3D(water);
Interactive example
Below is the complete code for adding a skybox and ocean to the scene.